*Post contains graphic content xD
Overview
Adafruit Industries’ SSD1331 organic light-emitting diode (OLED) display is an incredible little RGB screen that has quickly become my favorite in the category. I originally ordered it from HiLetgo when I was working on a watch-like device and needed a small user interface with decent resolution. The screen measures 0.9780″ x 0.8725″ from glass-edge to glass-edge while the actual display area, a 96 x 64 array of pixels, measures 0.8165″ x 0.535″ (thanks, digital caliper). The screen is normally marketed as being 0.95″ across so, yeah…buyer beware. Needless to say, the screen is small but it can display some killer eye-candy.
Setup
The setup is pretty simple and this YouTube video explains how to connect the pins. Wire it up like so (see sample code at the bottom of this page):
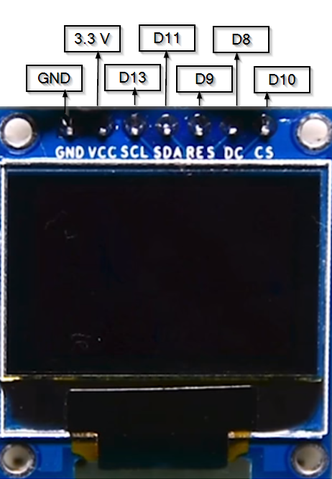
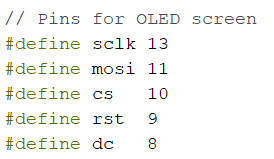
Add these line above your program’s setup() tag:
//Create OLED display object (can be called on to draw and display figures)
Adafruit_SSD1331 display = Adafruit_SSD1331(cs, dc, mosi, sclk, rst);
Start the display in your setup() tag:
display.begin();
And finally, add the Adafruit_GFX.h and Adafruit_SSD1331.h libraries to your sketch. See the bottom of this page for the full program.
Displaying Bitmaps
Using the Adafruit_GFX.h library, you can do a whole lot with the display.
Draw shapes (empty and filled)
Draw lines and place pixels
Customize text colors and sizes
Draw bitmaps
Given that this screen isn’t exactly a digital billboard, drawing fancy images for the world isn’t its primary purpose. Nonetheless, making the screen draw fancy images is unapologetically the topic of this post so strap in.
Here’s the quick and dirty version:
Create Yo’ Image
Download Pixelformer, a bitmap editor designed specifically for creating and modifying small, low-resolution images like icons
Once its installed, open it up and select File –> New
Select a screen size that matches your device (96 x 64 in this case) * Make sure that your dimensions are multiples of 8 (byte stuff)
Go Picasso on that mofo
Export the image as a Windows bitmap (.bmp file)
Convert Yo’ Image
Download LCD Assistant, a tool used to convert bitmaps into data arrays microcontrollers can understand
After its installed, open it and select File –> Load image
Select the .bmp file you just created
Change the Byte Orientation setting to “Horizontal”
Select File –> Save output (seriously, this is an in-and-out kind of thing)
Don’t try to save over another file since the type can get messed up (ex. saving over a PNG or JPEG file can cause some issues)
Load Yo’ Image
In your Arduino program, add the following line somewhere above your setup() code: const PROGMEM uint8_t bitMapName[] =
Open the new file using a text editor, like Notepad++
Copy everything from the first { to the last };
Back in Arduino add what you copied just after bitMapName[] (from step 1)
Show Yo’ Image
Within your loop(), add the line: drawBitmap(x, y, bitMapName, w, h, backColor, color); – x = x-position of upper left corner of image – y = y-position of upper left corner of image – bitMapName = your bit map name (o_o) – w = image width – h = image height – backColor = background pixel color – color = image pixel color *For the last two parameters, you can use Hex values from the color picker here. Define them at the top of your program like so and then use the color names. The downside to using this quick method is that your bitmap will be a binary one. Multicolor bitmaps can be displayed but that’s another topic.
Upload your program and enjoy what you have created
Sample Code
//Libraries for display
#include “Adafruit_GFX.h”
#include “Adafruit_SSD1331.h”
// Pins for OLED screen
#define sclk 13
#define mosi 11
#define cs 10
#define rst 9
#define dc 8
// Color definitions for OLED screen
#define BLACK 0x0000
#define BLUE 0x001F
#define RED 0xF800
#define GREEN 0x07E0
#define CYAN 0x07FF
#define MAGENTA 0xF81F
#define YELLOW 0xFFE0
#define WHITE 0xFFFF
//Create OLED display object (can be called on to draw and display figures)
Adafruit_SSD1331 display = Adafruit_SSD1331(cs, dc, mosi, sclk, rst);
const PROGMEM uint8_t mullr[]{
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xE1, 0xFF, 0xF0, 0xC0, 0xFC, 0x18, 0x1F, 0xF8,
0x1F, 0xF8, 0x01, 0xFF, 0xF8, 0xFF, 0xE3, 0xF3, 0xFF, 0x7E, 0x7F, 0xFE, 0x7F, 0xFE, 0x7C, 0x7F,
0xF8, 0xFF, 0xE3, 0xF3, 0xFF, 0x7E, 0x7F, 0xFE, 0x7F, 0xFE, 0x7F, 0x7F, 0xF8, 0x7F, 0xC3, 0xF3,
0xFF, 0x7E, 0x7F, 0xFE, 0x7F, 0xFE, 0x7F, 0x3F, 0xFA, 0x7F, 0xD3, 0xF3, 0xFF, 0x7E, 0x7F, 0xFE,
0x7F, 0xFE, 0x7F, 0x3F, 0xFA, 0x3F, 0x93, 0xF3, 0xFF, 0x7E, 0x7F, 0xFE, 0x7F, 0xFE, 0x7F, 0x3F,
0xFB, 0x3F, 0xB3, 0xF3, 0xFF, 0x7E, 0x7F, 0xFE, 0x7F, 0xFE, 0x7F, 0x3F, 0xFB, 0x1F, 0x33, 0xF3,
0xFF, 0x7E, 0x7F, 0xFE, 0x7F, 0xFE, 0x7C, 0x7F, 0xFB, 0x9F, 0x73, 0xF3, 0xFF, 0x7E, 0x7F, 0xFE,
0x7F, 0xFE, 0x01, 0xFF, 0xFB, 0x8F, 0x73, 0xF3, 0xFF, 0x7E, 0x7F, 0xFE, 0x7F, 0xFE, 0x67, 0xFF,
0xFB, 0xCE, 0xF3, 0xF3, 0xFF, 0x7E, 0x7F, 0xFE, 0x7F, 0xFE, 0x73, 0xFF, 0xFB, 0xC6, 0xF3, 0xF3,
0xFF, 0x7E, 0x7F, 0xFE, 0x7F, 0xFE, 0x79, 0xFF, 0xFB, 0xE4, 0xF3, 0xF3, 0xFF, 0x7E, 0x7F, 0xDE,
0x7F, 0xDE, 0x79, 0xFF, 0xFB, 0xE1, 0xF3, 0xF3, 0xFE, 0xFE, 0x7F, 0xDE, 0x7F, 0xDE, 0x7C, 0xFF,
0xFB, 0xF1, 0xF3, 0xF9, 0xFE, 0xFE, 0x7F, 0x9E, 0x7F, 0x9E, 0x7E, 0x7F, 0xFB, 0xF3, 0xF3, 0xFC,
0xF9, 0xFE, 0x7F, 0x3E, 0x7F, 0x3E, 0x7F, 0x1F, 0xE0, 0xFB, 0xC0, 0xFF, 0x07, 0xF8, 0x00, 0x38,
0x00, 0x38, 0x1F, 0x07, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF,
0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF, 0xFF
};
void setup(void)
{
//Start communication on Serial (USB)
Serial.begin(9600);
//Start OLED display, clear anything that may have previously been shown, and draw bitmap
display.begin();
display.fillScreen(BLACK);
display.drawBitmap(0,0,mullr,96,64,RED,BLACK);
}
void loop(void)
{
//Sample code. Bitmap is drawn in setup()
}
Comments